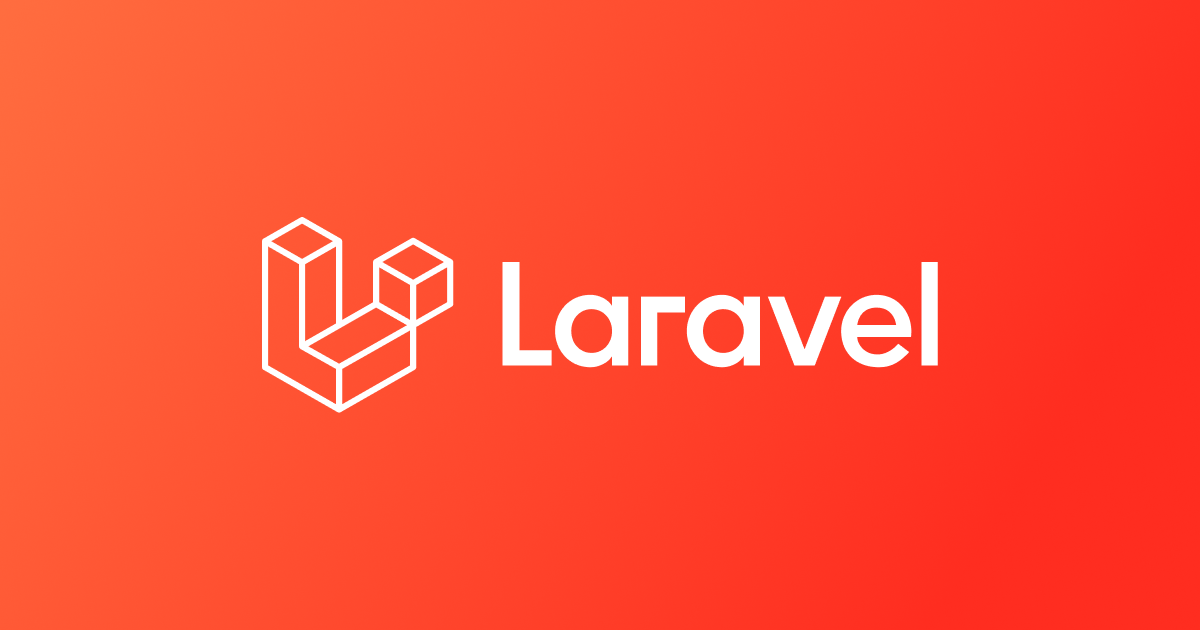
Laravel: Get Start to Build Powerful Web Applications
Laravel is a popular PHP framework that has gained immense popularity among developers for building web applications. It was created by Taylor Otwell in 2011 and has since become one of the leading frameworks in the PHP ecosystem. Laravel provides a robust and elegant way to develop web applications, with a focus on simplicity, elegance, and code readability.
Why Choose Laravel?
There are several reasons why developers choose Laravel for their web development projects:
Elegant Syntax: Laravel follows the principle of "expressive syntax" which means the code is easy to read and write. It provides a clean and elegant syntax that allows developers to express their ideas without any unnecessary clutter.
Modularity: Laravel follows the modular approach, which means it is built on a set of individual and independent libraries. This modular architecture allows developers to use only the components they need and keeps the application codebase clean and maintainable.
MVC Architecture: Laravel follows the Model-View-Controller (MVC) architectural pattern, which provides a clear separation of concerns. The model represents the data and business logic, the view handles the presentation logic, and the controller manages the flow between the model and view. This separation enhances code organization and maintainability.
Database Migration: Laravel provides a powerful database migration system that allows developers to define and manage database schemas using PHP code instead of SQL. This makes it easy to version control database changes and collaborate with other developers.
ORM (Object-Relational Mapping): Laravel comes with an intuitive ORM called Eloquent, which provides an easy and expressive way to interact with databases. It allows developers to work with database records as objects, making database operations more natural and less error-prone.
Routing and Middleware: Laravel provides a clean and simple routing system that allows developers to define application routes and map them to appropriate controllers. It also includes middleware, which enables developers to add additional layers of logic to the request/response lifecycle.
Caching and Performance Optimization: Laravel offers built-in support for various caching mechanisms, including file caching, database caching, and in-memory caching with popular backends like Redis. It also includes features like query caching and route caching, which can significantly improve application performance.
Security: Laravel takes security seriously and includes features like protection against cross-site scripting (XSS) attacks, cross-site request forgery (CSRF) protection, and encrypted password hashing out of the box. It also provides tools for handling authentication and authorization.
Why Laravel is an Excellent Choice for Beginners
When it comes to web development, choosing the right framework can make a significant impact on your learning journey, especially if you're a beginner. Laravel, the PHP framework created by Taylor Otwell, has gained immense popularity in recent years and has become a go-to choice for developers of all skill levels. However, it particularly stands out as an excellent choice for beginners. In this section, we will explore why Laravel is so well-suited for those who are just starting their web development journey.
Clear and Concise Documentation
Laravel boasts exceptional documentation that is well-structured, comprehensive, and beginner-friendly. The documentation provides detailed explanations of Laravel's features, concepts, and usage, making it easier for beginners to understand and grasp the framework's fundamentals. Laravel's documentation covers everything from installation and configuration to advanced topics like authentication, database management, and testing. With its clear examples and step-by-step guides, beginners can quickly learn how to use Laravel effectively.
Expressive and Elegant Syntax
One of the standout features of Laravel is its expressive and elegant syntax. The framework leverages modern PHP features to provide a clean and intuitive syntax that is easy to read and understand. Laravel's syntax emphasizes simplicity and clarity, allowing beginners to write code that is both concise and maintainable. This approach reduces the learning curve for beginners and helps them focus on building their applications rather than getting lost in complex syntax or cumbersome boilerplate code.
Comprehensive Feature Set
Laravel comes equipped with a wide array of features that simplify common web development tasks. These features are designed to streamline the development process and promote best practices. From routing and middleware to database management and authentication, Laravel provides a comprehensive set of tools that beginners can leverage to build robust and secure web applications. Having these features readily available allows beginners to focus on learning core concepts and quickly create functional applications without reinventing the wheel.
MVC Architecture
Laravel follows the Model-View-Controller (MVC) architectural pattern, which provides a structured and organized approach to web development. The MVC pattern separates the application's logic into three distinct components: models, views, and controllers. This separation of concerns enhances code maintainability, scalability, and reusability. Beginners can leverage Laravel's built-in support for MVC to develop well-structured applications that are easy to understand and maintain.
Laravel Ecosystem and Community Support
Laravel has a thriving ecosystem and a supportive community that significantly contributes to its growth and development. The ecosystem consists of various packages, libraries, and extensions that extend Laravel's functionality. These resources provide beginners with a wealth of options to enhance their applications and solve specific problems more efficiently. Additionally, the Laravel community is known for its active and helpful nature. Beginners can seek guidance, ask questions, and find support through Laravel's official forums, community-driven websites, and social media channels.
Learning Resources and Tutorials
Another reason why Laravel is an excellent choice for beginners is the abundance of learning resources available. There are numerous tutorials, video courses, and blog posts dedicated to teaching Laravel from scratch. These resources cater specifically to beginners and provide step-by-step instructions and hands-on examples to help them grasp the framework's concepts effectively. The availability of high-quality learning materials ensures that beginners can find the guidance they need to get started with Laravel.
Getting Started with Laravel
To get started with Laravel, you'll need to have PHP installed on your machine. You can download the latest version of PHP from the Official PHP Website. Once you have PHP installed, you can install Laravel using Composer, the dependency manager for PHP. You can review my What is Composer and How to Install it? (Mac, Linux, and Windows) article to learn how to install Composer.
Open a terminal or command prompt and run the following command to install Laravel globally:
composer global require laravel/installer
After the installation is complete, you can create a new Laravel project by running the following command:
laravel new myproject
This will create a new Laravel project named "myproject" in the current directory. You can then navigate to the project directory and start the development server:
cd myproject
php artisan serve
Now, you can access your Laravel application by visiting http://localhost:8000 in your web browser.
Laravel Code Examples
Routing
Routing is an essential part of any web application, and Laravel provides a simple and expressive way to define routes. Here's an example of defining a basic route in Laravel:
Route::get('/welcome', function () {
return 'Welcome to Laravel!';
});
In this example, we define a GET
route that maps to the /welcome
URL. When a user visits that URL, the anonymous function will be executed, and the message "Welcome to Laravel!" will be returned.
You can also define routes with parameters:
Route::get('/user/{id}', function ($id) {
return 'User ID: ' . $id;
});
In this case, the {id}
parameter captures the dynamic part of the URL and passes it to the callback function.
Controllers
Controllers are responsible for handling the application logic and processing requests. They provide a structured way to organize your code and separate it from the routes. Here's an example of a basic controller in Laravel:
namespace App\Http\Controllers;
use App\Models\User;
class UserController extends Controller
{
public function show($id)
{
$user = User::find($id);
return view('user.show', ['user' => $user]);
}
}
In this example, we define a UserController
that has a show
method. The show
method retrieves a user by their ID from the database and passes it to the user.show
view.
Views and Blade Templating Engine
Laravel uses the Blade templating engine to render views. Blade provides a concise and expressive syntax for writing templates. Here's an example of a Blade template:
<html>
<head>
<title>User Profile</title>
</head>
<body>
<h1>User Profile</h1>
<p>Name: {{ $user->name }}</p>
<p>Email: {{ $user->email }}</p>
</body>
</html>
In this example, we use double curly braces {{ }}
to output dynamic data within the HTML markup.
Database Operations with Eloquent
Laravel's Eloquent ORM simplifies database operations by providing an intuitive syntax for working with database records. Here's an example of creating a new record using Eloquent:
$user = new User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->save();
In this example, we create a new User
instance, set the name
and email
properties, and then call the save
method to persist the record to the database.
Authentication and Authorization
Laravel provides a robust authentication system out of the box. You can easily add user registration, login, and password reset functionality to your application. Here's an example of authenticating a user:
if (Auth::attempt(['email' => $email, 'password' => $password])) {
// Authentication successful
} else {
// Authentication failed
}
In this example, we use the attempt
method to validate the user's credentials. If the credentials are valid, the user will be authenticated.
Laravel also includes authorization features, allowing you to define access control policies and restrict certain actions based on user roles and permissions.
Conclusion
Laravel is a powerful PHP framework that simplifies web development and provides a wide range of features and tools. It promotes clean and maintainable code through its elegant syntax, modular architecture, and adherence to the MVC pattern. With Laravel, you can build robust web applications quickly and efficiently, thanks to its built-in features like routing, middleware, database migration, ORM, caching, and security mechanisms. By using Laravel, developers can focus on their application's logic and functionality without getting bogged down by repetitive tasks and low-level implementation details.
Furthermore, Laravel has a thriving community that actively contributes to its development and provides a vast ecosystem of packages and extensions. This makes it easier for developers to leverage existing solutions and integrate third-party libraries into their Laravel projects.
In conclusion, Laravel is an excellent choice for PHP web development due to its simplicity, elegance, and extensive feature set. Whether you're building a small personal website or a large-scale enterprise application, Laravel provides the tools and structure you need to develop high-quality and maintainable code.
Remember, this article only scratches the surface of what Laravel has to offer. To fully explore the capabilities of Laravel, I encourage you to visit the Official Laravel Website and dive into the extensive documentation and tutorials available there.